Now we have a list, lets create a detail view for each note. First, create a new view controller (File>New>New File>Cocoa Touch>Objective-C Class) named NoteDetailViewController that is a subclass of UIViewController. Next, drag out a new View Controller in Interface Builder, give it the custom class NoteDetailViewController and add 2 labels and a textview (make sure it is not editable by clicking the appropriate box in the object properties). Open the assistant editor and make sure NoteDetailViewController.m is shown in the right hand frame. Wire up the new storyboard objects by ctrl dragging from each object to the NoteDetailViewController.m and name them titleLabel, dateLabel, and contentView. Finally, create a push manual segue by ctrl dragging from NotesListViewController to NoteDetailViewController and name it listToNote. You should now have the following:
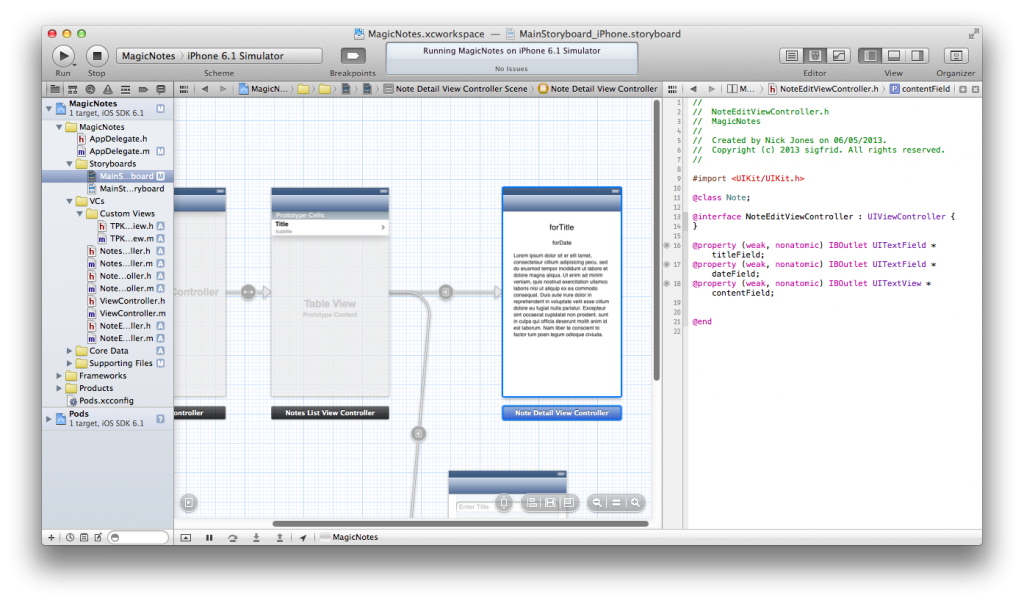
Go back to NoteDetailViewController.m and replace it with:
#import "NoteDetailViewController.h" #import "Note+Create.h" @interface NoteDetailViewController () @property (nonatomic, strong) Note *note; @end @implementation NoteDetailViewController @synthesize note = _note; - (id)initWithNibName:(NSString *)nibNameOrNil bundle:(NSBundle *)nibBundleOrNil { self = [super initWithNibName:nibNameOrNil bundle:nibBundleOrNil]; if (self) { } return self; } - (void)viewDidLoad { [super viewDidLoad]; // Do any additional setup after loading the view. self.titleLabel.text = self.note.title; self.contentView.text = self.note.content; // Convert date object to desired output format self.dateLabel.text =[self.note formatDateAsString]; } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; } @end
Finally, we just need to get the table view controller to pass the correct note to our detail view controller. Selecting a table row triggers the segue we added in Interface Builder, and also passes the indexPath of the selected cell.
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath { [tableView deselectRowAtIndexPath:indexPath animated:YES]; [self performSegueWithIdentifier:@"listToNote" sender:indexPath]; }
We pass a note to the detail view controller by checking that the destination controller responds to setNote. If it does we use this method with the note found at the indexPath that was passed as sender. We haven’t explicitly defined note setter ‘setNote’ in the target NoteDetailViewController, but it has been generated automatically by Xcode when we synthesized the note instance variable.
#pragma mark Segue -(void) prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender { if ([segue.destinationViewController respondsToSelector:@selector(setNote:)]){ [segue.destinationViewController performSelector:@selector(setNote:) withObject:[self.fetchedResultsController objectAtIndexPath:sender]]; } }
Now you can run the app, and hopefully clicking any row should show you more note details.